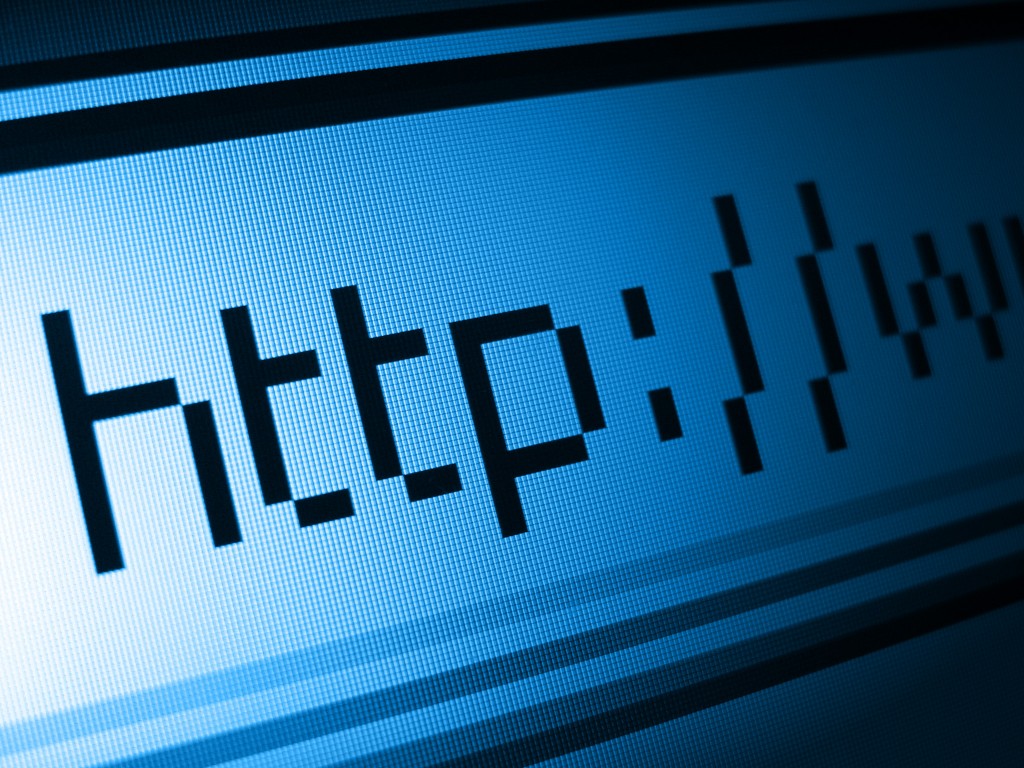
Why rest-assured doesn't redirect POST requests ?
If you guys like testing API's like i do, then you might face with issue when you try to make a POST request with rest-assured and expect to be redirected to another URL. If you read documentation before, then you would probably try one of this things :
- For a specific request:
given().config(RestAssured.config().redirect(redirectConfig().followRedirects(false)))
- or using a RequestSpecBuilder:
RequestSpecification spec = new RequestSpecBuilder().setConfig(RestAssured.config().redirect(redirectConfig().followRedirects(false))).build();
- or for all requests:
RestAssured.config = config().redirect(redirectConfig().followRedirects(true).and().maxRedirects(0));
But non of this will work for one specific reason - HTTP Specification, which states :
If the 302 status code is received in response to a request other than GET or HEAD, the user agent MUST NOT automatically redirect the request unless it can be confirmed by the user, since this might change the conditions under which the request was issued.
Which means that there's no bug or misleading behaviour in rest-assured. This is how things should actually work. In order to overcome this issue you could :
- Ask developers to return 303 code as a redirect for POST code
- Change POST call to Get and then redirect will work properly
- Or you could manually redirect yourself by:
- taking
Location
from response of the first call - Merging cookies from one request to another
- Make new request with needed headers\cookies to a extracted location and then verifying that redirect intention was correct.
As an example of code :
private Response findToken(Response response) {
while (response.cookie("at") == null) {
logger.logResponse(response, "Find Token");
cookies = mergeCookies(cookies, response.detailedCookies());
String url = response.then().extract().header("Location");
if (url == null) {
logger.error("Url for parse is null.");
throw new IllegalStateException("Url for parse is null.");
}
Map<String, String> params = parseParamsFromUrl(url);
response = with().spec(new RequestSpecBuilder()
.setBaseUri(url)
.addParams(params)
.setConfig(RestAssuredConfig.newConfig().redirect(RedirectConfig.redirectConfig().followRedirects(false)))
.addCookies(cookies)
.addHeader(HttpHeaders.ACCEPT, "text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8")
.build())
.get();
}
return response;
}
Thanks to @crazyk2
from automated-info.com
I was able to find this answer thanks to a nice blog post from Johan Haleby