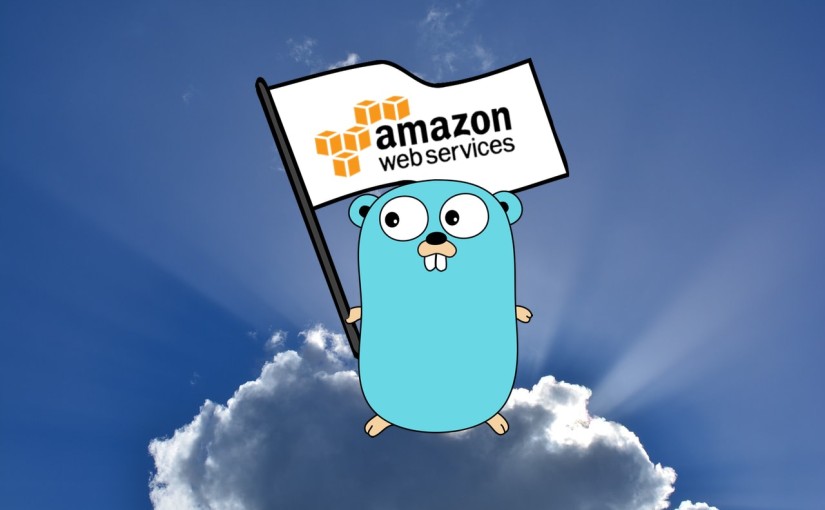
Fixing AWS "not authorized to perform: ssm:GetParameter" error in Go lambda
So, i started to learn Go and decided to write some chatbot that for our Slack in order to ask for some routine tasks that we do during testing or development in New10. Before we were using a lot of javascript and serverless, so i took some code snippets from previous projects in order to comply with out standarts and to speed things up.
My parameter-store
policy looked like this :
parameter-store:
PolicyName: ${self:custom.resourcePrefix}-parameter-store
PolicyDocument:
Version: "2012-10-17"
Statement:
- Effect: Allow
Action:
- ssm:GetParameters
Resource:
- arn:aws:ssm:${self:custom.region}:${self:custom.stage.infra.AWS_ACCOUNT}:parameter/handybot-service.*
I have implemented a simple function in go that would just fetch some parameter from AWS Parameter store :
func getParameter(parameterName string) (string, error) {
region := "eu-west-1"
sess, err := session.NewSessionWithOptions(session.Options{
Config: aws.Config{Region: aws.String(region)},
SharedConfigState: session.SharedConfigEnable,
})
if err != nil {
log.Fatal(err)
return "", nil
}
ssmsvc := ssm.New(sess, aws.NewConfig().WithRegion(region))
withDecryption := false
param, err := ssmsvc.GetParameter(&ssm.GetParameterInput{
Name: ¶meterName,
WithDecryption: &withDecryption,
})
if err != nil {
return "", err
}
value := *param.Parameter.Value
return value, nil
}
so calling it from my main function would be as easy as :
value, paramStoreErr := getParameter("handybot-service.BLA")
log.Printf("parameter name is %s, %s ", value, paramStoreErr)
But, when i tried that, i got :
AccessDeniedException: User: arn:aws:sts::{aws_account_number}:assumed-role/sls-dev-handybot-LambdaRoleNoVpc/
sls-dev-handybot-say-hello is not authorized to perform: ssm:GetParameter on resource: arn:aws:ssm:eu-west-1:
{aws_account_number}:parameter/handybot-service.BLA status code: 400, request
id: c299900c-5567-44b0-aa19-e1c57930e0f9: requestError null
After checking everything multiple times, i have found this post .
It appears that you need to specificly add - ssm:GetParameter
action to your policy in order for this to work. It seem that aws documentation was not clearly pointing to that. So, after i have updated my policy to this :
parameter-store:
PolicyName: ${self:custom.resourcePrefix}-parameter-store
PolicyDocument:
Version: "2012-10-17"
Statement:
- Effect: Allow
Action:
- ssm:GetParameters
- ssm:GetParameter
Resource:
- arn:aws:ssm:${self:custom.region}:${self:custom.stage.infra.AWS_ACCOUNT}:parameter/handybot-service.*
it all worked! I hope this will help you guys as well.